Resolving Command Parameters into Input Types
Internal editing note: Needs further edits to include valid JSON examples of all supported input type. See https://bitbucket.org/xnatdev/container-service/src/master/docs/command.md#input-types and track work in https://radiologics.atlassian.net/browse/XDOC-343 .
In order to power the Container Launch UI, we will need to take any user-settable parameters and convert them into HTML form elements. If not specified, a text input will be used by default. However, many parameter types will benefit from a fine-grained input styling. It's worth noting that, in addition to the "name" and "value" properties, HTML inputs should also have a "label" and can also make use of helptext to guide the user. Enumerated lists and dropdown menus have additional label properties for each value option.
Therefore, a JSON input definition that looks like this:
a_parent_input: {
type: "select-one",
name: "inputName",
description: "This is a description of this input",
user_settable: true,
advanced: false,
required: true,
parent: null,
values: {
default: [
{ value: "parent_value1", label: "parent value 1" } ,
{ value: "parent_value2", label: "parent value 2" }
]
}
}
Would resolve into this:
<div data-name="inputName" class="panel-element">
<label class="element-label" for="inputName">Select An Option</label>
<div class="element-wrapper">
<label>
<select id="input-name" name="inputName" title="inputName" class="required">
<option value="option1">Option 1</option>
<option value="option2">Option 2</option>
</select>
</label>
<div class="description">This is helptext</div>
</div>
<div class="clear"></div>
</div>
And this would be rendered as part of a configuration modal like so:
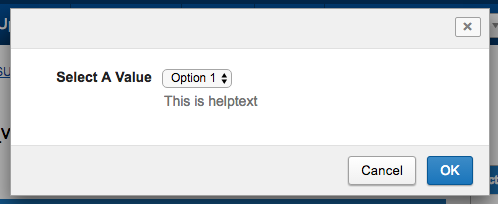
Usage Notes
Any parameter that must be passed to the command but is not considered user-settable should be specified in a hidden input.
Hidden parameters can also be accompanied by a static form control, which displays the value that is being passed, without letting the user change that value.
Default Input Types and Base HTML Elements
default / text / integer / float
<label for="NAME">LABEL</label>
<input type="text" name="NAME" value="VALUE" />
Required | Optional |
---|---|
|
|
Select-one
<label for="NAME">LABEL</label>
<select name="NAME">
<option value="OPTIONVAL">OPTIONLABEL</option>
</select>
Required | Optional |
---|---|
option(s):
|
option(s):
|
Select-many (Multiselect)
<label for="NAME">LABEL</label>
<label><input type="checkbox" name="NAME" value="OPTIONVAL" />OPTIONLABEL</label>
Required | Optional |
---|---|
option(s):
|
option(s):
|
Boolean
<label for="NAME">LABEL</label>
<label><input type="radio" name="NAME" value="true" />ONTEXT</label>
<label><input type="radio" name="NAME" value="false" />OFFTEXT</label>
Required | Optional |
---|---|
|
|
Hidden
<input type="hidden" name="NAME" value="VALUE" />
Required | Optional |
---|---|
|
Static
<label>LABEL</label>
<span>VALUE</span>
<input type="hidden" name="NAME" value="VALUE" />
Required | Optional |
---|---|
|
StaticList
(Used to display a hidden list of inputs, such as preselected scans.)
<label>LABEL</label>
<ul>
<li>VALUE</li>
</ul>
<input type="hidden" name="NAME[]" value="VALUE" />
Required | Optional |
---|---|
|